Struts 1.x Fisrt Example
Complete
web MVC( Model - View - Controller ) framework, provides complete web
form components, validator, internalization, error handling, tiles
layout. Struts 1.x is low learning curve and easy to implement it.
Step
- 1.0 Create Directory Structure
Create
the folder structure and add struts libraries under the lib folder
which is inside the WEB-INF.
Step
- 2.0 Create Action Form
Create
a java class called “FirstExampleForm.java” by extending struts
Action Form. This is use to hold the data later.
package
first.form;
import
java.io.Serializable;
import
org.apache.struts.action.ActionForm;
public
class
FirstExampleForm extends
ActionForm implements
Serializable{
/**
*
*/
private
static
final
long
serialVersionUID
= 1L;
private
String message;
public
String getMessage() {
return
message;
}
public
void
setMessage(String message) {
this.message
= message;
}
}
Step
- 3.0 Create Action Class
Create
a java class called “FirstExampleAction.java” by extending struts
Action (Action Controller). Struts forward the request by override
the execute() method.
package
first.action;
import
javax.servlet.http.HttpServletRequest;
import
javax.servlet.http.HttpServletResponse;
import
org.apache.struts.action.Action;
import
org.apache.struts.action.ActionForm;
import
org.apache.struts.action.ActionForward;
import
org.apache.struts.action.ActionMapping;
import
first.form.FirstExampleForm;
public
class
FirstExampleAction extends
Action {
public
ActionForward execute(ActionMapping actionMapping, ActionForm form,
HttpServletRequest
request, HttpServletResponse response)
throws
Exception{
FirstExampleForm
firstExampleForm = (FirstExampleForm)form;
firstExampleForm.setMessage("First
Example of Struts is Success");
return
actionMapping.findForward("success");
}
}
Step
- 4.0 Create JSP view page
Create
a jsp called “FirstExample.jsp” This access the Action Form
object via Struts tag library and print the Form properties.
<%@
page language="java"
contentType="text/html;
charset=UTF-8" pageEncoding="UTF-8"%>
<%@taglib
uri="http://struts.apache.org/tags-bean"
prefix="bean"%>
<!DOCTYPE
html PUBLIC
"-//W3C//DTD HTML 4.01 Transitional//EN"
"http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta
http-equiv="Content-Type"
content="text/html;
charset=UTF-8">
<title>First
Example</title>
</head>
<body>
<h3><bean:write
name="FirstExampleForm"
property="message"/></h3>
</body>
</html>
Step
- 5.0 Create struts-config.xml
Create
a struts-config.xml file for the Struts configuration details, and
put it into the WEB-INF/config folder.
Define
the form bean name (FirstExampleForm).
Defien
the Action mapping controller (FirstExampleAction),
match the /firstExample
web path to FirstExampleAction.
<?xml
version="1.0"
encoding="UTF-8"?>
<!DOCTYPE
struts-config PUBLIC
"-//Apache
Software Foundation//DTD Struts Configuration 1.3//EN"
"http://jakarta.apache.org/struts/dtds/struts-config_1_3.dtd">
<struts-config>
<form-beans>
<form-bean
name="FirstExampleForm"
type="first.form.FirstExampleForm"/>
</form-beans>
<action-mappings>
<action
path="/firstExample"
type="first.action.FirstExampleAction"
name="FirstExampleForm">
<forward
name="success"
path="/WEB-INF/jsp/FirstExample.jsp"/>
</action>
</action-mappings>
</struts-config>
Step
- 6.0 The web application deployment descriptor ( web.xml )
In
web.xml file, configure the Struts ActionServlet instance and map it
with url-pattern “*.do”, so that the container is aware of all
the “*.do” pattern will redirect to Struts ActionServlet.
<?xml
version="1.0"
encoding="UTF-8"?>
<!DOCTYPE
struts-config PUBLIC
"-//Apache
Software Foundation//DTD Struts Configuration 1.3//EN"
"http://jakarta.apache.org/struts/dtds/struts-config_1_3.dtd">
<struts-config>
<form-beans>
<form-bean
name="FirstExampleForm"
type="first.form.FirstExampleForm"/>
</form-beans>
<action-mappings>
<action
path="/firstExample"
type="first.action.FirstExampleAction"
name="FirstExampleForm">
<forward
name="success"
path="/WEB-INF/jsp/FirstExample.jsp"/>
</action>
</action-mappings>
</struts-config>
Step
- 7.0 Run the application on server and out put will be-
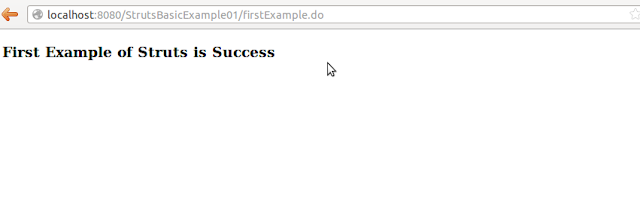