Struts 1.x Dispatch Action Example
DispatchAction
provides a mechanism for grouping a set of related functions into a
single action. This avoid by creating separate actions for each
functions. In this example lets see how to group a set of related actions like add and del into a
single action called DispatchObjectAction.
The
class DispatchAction extends
org.apache.struts.actions.DispatchAction. This class does not provide
an implementation of the execute() method as the normal Action class
does. The DispatchAction uses the execute method to manage delegating
the request to the individual methods based on the incoming request
parameter. For example if the incoming parameter is "method=add",
then the add method will be invoked. These methods should have
similar signature as the execute method.
Step
- 1.0 Create Directory Structure
Create
the folder structure and add struts libraries under the lib folder
which is inside the WEB-INF.
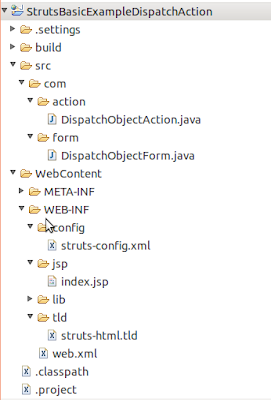
Step
- 2.0 Create Action Form
Create
a java class called “DispatchObjectForm.java” by extending struts
Action Form. This is use to hold the data later.
package
com.form;
import
java.io.Serializable;
import
org.apache.struts.action.ActionForm;
public
class
DispatchObjectForm extends
ActionForm implements
Serializable{
/**
*
*/
private
static
final
long
serialVersionUID
= 1L;
private
String actionName;
private
String message;
public
String getActionName() {
return
actionName;
}
public
void
setActionName(String actionName) {
this.actionName
= actionName;
}
public
String getMessage() {
return
message;
}
public
void
setMessage(String message) {
this.message
= message;
}
}
Step
- 3.0 Create Action Class
Create
a class called “DispatchObjectAction.java” by extending the
DispatchAction. The DispatchAction uses the execute method to manage
delegating the request to the individual methods based on the
incoming request parameter.
package
com.action;
import
javax.servlet.http.HttpServletRequest;
import
javax.servlet.http.HttpServletResponse;
import
org.apache.struts.action.ActionForm;
import
org.apache.struts.action.ActionForward;
import
org.apache.struts.action.ActionMapping;
import
org.apache.struts.actions.DispatchAction;
import
com.form.DispatchObjectForm;
public
class
DispatchObjectAction extends
DispatchAction{
public
ActionForward add(ActionMapping mapping, ActionForm form,
HttpServletRequest
request, HttpServletResponse response)throws
Exception{
DispatchObjectForm
objectForm = (DispatchObjectForm)form;
objectForm.setActionName("Add");
objectForm.setMessage("Inside
the Add Dispatch");
return
mapping.findForward("success");
}
public
ActionForward del(ActionMapping mapping, ActionForm form,
HttpServletRequest
request, HttpServletResponse response)throws
Exception{
DispatchObjectForm
objectForm = (DispatchObjectForm)form;
objectForm.setActionName("Delete");
objectForm.setMessage("Inside
the del Dispatch");
return
mapping.findForward("success");
}
}
Step
- 4.0 Create JSP view page
Create
a jsp called “index.jsp” This access the Action Form object via
Struts tag library and print the Form properties.
<%@
page
language="java"
contentType="text/html;
charset=UTF-8"
pageEncoding="UTF-8"%>
<%@
taglib
uri="/WEB-INF/tld/struts-html.tld"
prefix="html"%>
<%@taglib
uri="http://struts.apache.org/tags-bean"
prefix="bean"%>
<!DOCTYPE
html PUBLIC
"-//W3C//DTD HTML 4.01 Transitional//EN"
"http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta
http-equiv="Content-Type"
content="text/html;
charset=UTF-8">
<title>Dispatch
Action Example</title>
</head>
<body>
<html:form
action="DispatchAction"
method="add">
<table>
<tr><td
colspan="2">
<bean:write
name="DispatchObjectForm"
property="actionName"/>
</td></tr>
<tr><td
colspan="2">
<bean:write
name="DispatchObjectForm"
property="message"/>
</td></tr>
<tr>
<td><html:submit
property="method"
value="add"/></td>
<td><html:submit
property="method"
value="del"/></td>
</tr>
</table>
</html:form>
</body>
</html>
Step
- 5.0 Create struts-config.xml
Create
a struts-config.xml file for the Struts configuration details, and
put it into the WEB-INF/config folder.
Define
the form bean name (FirstExampleForm).
Defien
the Action mapping controller (FirstExampleAction),
match the /firstExample
web path to FirstExampleAction.
<?xml
version="1.0"
encoding="UTF-8"?>
<!DOCTYPE
struts-config PUBLIC
"-//Apache
Software Foundation//DTD Struts Configuration 1.3//EN"
"http://jakarta.apache.org/struts/dtds/struts-config_1_3.dtd">
<struts-config>
<form-beans>
<form-bean
name="DispatchObjectForm"
type="com.form.DispatchObjectForm"/>
</form-beans>
<action-mappings>
<action
input="/WEB-INF/jsp/index.jsp"
parameter="method"
name="DispatchObjectForm"
path="/DispatchAction"
type="com.action.DispatchObjectAction">
<forward
name="success"
path="/WEB-INF/jsp/index.jsp"/>
</action>
</action-mappings>
</struts-config>
Step
- 6.0 The web application deployment descriptor ( web.xml )
In
web.xml file, configure the Struts ActionServlet instance and map it
with url-pattern “*.do”, so that the container is aware of all
the “*.do” pattern will redirect to Struts ActionServlet.
<?xml
version="1.0"
encoding="UTF-8"?>
<web-app
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="http://java.sun.com/xml/ns/javaee"
xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee
http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd"
id="WebApp_ID"
version="2.5">
<display-name>StrutsBasicExampleDispatchAction</display-name>
<servlet>
<servlet-name>action</servlet-name>
<servlet-class>
org.apache.struts.action.ActionServlet
</servlet-class>
<init-param>
<param-name>config</param-name>
<param-value>/WEB-INF/config/struts-config.xml</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>action</servlet-name>
<url-pattern>*.do</url-pattern>
</servlet-mapping>
</web-app>
Step
- 7.0 Run the application on server and out put will be