Struts
1.x Struts Tiles Framework Example
In
this example we declare the “TilesPlugin” plug-in class
in the struts configuration file.
<!--
Tiles plug-in -->
<plug-in
className="org.apache.struts.tiles.TilesPlugin">
<set-property
property="definitions-config"
value="/WEB-INF/config/tiles-defs.xml"
/>
</plug-in>
We
need to defines the tiles mapping on the
tiles-defs.xml.
In
this example you will find out below tiles and jsp tags.
<definition
name="main.layout"
path="/WEB-INF/jsp/main.jsp">
<put
name="header"
value="/WEB-INF/jsp/header.jsp"/>
<put
name="body"
value="/WEB-INF/jsp/body.jsp"/>
<put
name="footer"
value="/WEB-INF/jsp/footer.jsp"/>
</definition>
<definition
name="login.welcome.page"
extends="main.layout">
<put
name="body"
value="/WEB-INF/jsp/welcome.jsp"/>
</definition>
<tiles:insert
attribute="body"></tiles:insert>
<jsp:include
page="footer.jsp"></jsp:include>
Step
- 1.0 Create Directory Structure
Create
the folder structure and add struts libraries under the lib folder
which is inside the WEB-INF.
If
plan to call the tld from the project itself then add the tlds to the
WEB-INF.
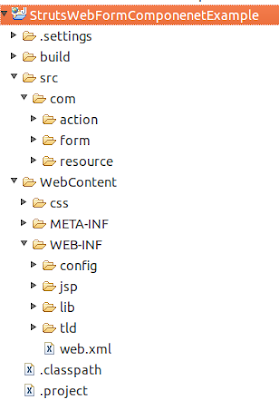
Step
- 2.0 Create Action Form
Create
a java class called “LoginForm
.java” by extending struts Action Form. This is use
to hold the data later. Also this example we use the form validation
by using ActionErrors validate() method.
package
com.form;
import
java.io.Serializable;
import
javax.servlet.http.HttpServletRequest;
import
org.apache.struts.action.ActionErrors;
import
org.apache.struts.action.ActionForm;
import
org.apache.struts.action.ActionMapping;
import
org.apache.struts.action.ActionMessage;
import
org.apache.struts.action.ActionMessages;
public
class
LoginForm extends
ActionForm implements
Serializable {
/**
*
*/
private
static
final
long
serialVersionUID
= 1L;
private
String username;
private
String password;
private
String hiddenpassword;
//TODO
generate getter and setter methods ...
public
ActionErrors validate(ActionMapping mapping, HttpServletRequest
request){
ActionErrors
errors = new
ActionErrors();
if(null
== getUsername() || getUsername().compareToIgnoreCase("")
== 0){
ActionMessages
messages = new
ActionMessages();
messages.add("display.error.username",
new
ActionMessage("error.username.required"));
errors.add(messages);
}
if(null
== getPassword() || getPassword().compareToIgnoreCase("")
== 0){
ActionMessages
messages = new
ActionMessages();
messages.add("display.error.password",
new
ActionMessage("error.password.required"));
errors.add(messages);
}
return
errors;
}
public
void
resert(ActionMapping mapping, HttpServletRequest request){
this.username
= "";
this.password
= "";
}
}
Step
- 3.0 Create Action Class
Create
a class called “LoginAction
.java” by extending the Action.
package
com.action;
import
javax.servlet.http.HttpServletRequest;
import
javax.servlet.http.HttpServletResponse;
import
org.apache.struts.action.Action;
import
org.apache.struts.action.ActionForm;
import
org.apache.struts.action.ActionForward;
import
org.apache.struts.action.ActionMapping;
public
class
LoginAction extends
Action {
public
ActionForward execute(ActionMapping mapping, ActionForm form,
HttpServletRequest
request, HttpServletResponse response) throws
Exception{
return
mapping.findForward("success");
}
}
Step
- 4.0 Create JSP view page
Create
jsp called “main.jsp” and “mainDetails.jsp”. Other than that
create a resource file to call the property values (
common.properties ) and create a css files to call the styles (
myStyles.css ).
4.1
main.jsp -
<%@
page language="java"
contentType="text/html;
charset=UTF-8" pageEncoding="UTF-8"%>
<%@
taglib
uri="/WEB-INF/tld/struts-html.tld"
prefix="html"%>
<%@
taglib
uri="http://struts.apache.org/tags-bean"
prefix="bean"%>
<%@
taglib
uri="http://struts.apache.org/tags-tiles"
prefix="tiles"
%>
<!DOCTYPE
html PUBLIC
"-//W3C//DTD HTML 4.01 Transitional//EN"
"http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta
http-equiv="Content-Type"
content="text/html;
charset=UTF-8">
<title>title</title>
<link
rel="stylesheet"
href="<%=request.getContextPath()%>/css/myStyles.css">
</head>
<body>
<div
style="width:100%">
<div
id="header"
style="width:100%">
<jsp:include
page="header.jsp"></jsp:include>
</div>
<div
id="body"
style="width:100%">
<tiles:insert
attribute="body"></tiles:insert>
</div>
<div
id="footer"
style="width:100%;height:15px;">
<jsp:include
page="footer.jsp"></jsp:include>
</div>
</div>
</body>
</html>
4.2
header.jsp -
<div
style="padding:16px;">
<div
class="divOuterTable">
<div
class="divOuterRow">
<div
class="divColumn">
<div
align="center"><img
src="<%=request.getContextPath()%>/images/index.jpeg"
border="0"
height="180"
width="180"
align="left"/></div>
</div>
<div
class="divColumn">
<div
align="right"><h2>Skilled
Migrant
Category</h2></div>
<div
align="right"><h3>User
Application System</h3></div>
<div
align="right"><b>---------------------------------------</b></div>
</div>
<div
class="divOuterRow">
<div
class="divColumn"> </div>
<div
class="divColumn">
<div
align="right"
class="h3font">Guide
/ User Registration Form</div>
<div
align="right"
class="h3font">255
A, Main Street, Colombo
01, Sri
Lanka</div>
<div
align="right"
class="h3font">+94
123987643 | +94 770985824</div>
</div>
</div>
</div>
</div>
</div>
4.3
header.jsp -
<div
class="divOuterTable">
<div
class="divOuterRow">
<div
class="divOuterColumn">
<div
style="padding-top:2;padding-bottom:2;background-color:gray;color:white;">
<h5> For
further information on immigration visit
www.immigrationsrilanka.com</h5>
</div>
</div>
</div>
</div>
4.4
login.jsp -
<%@
page
language="java"
contentType="text/html;
charset=UTF-8"
pageEncoding="UTF-8"%>
<%@
taglib
uri="/WEB-INF/tld/struts-html.tld"
prefix="html"%>
<%@
taglib
uri="http://struts.apache.org/tags-bean"
prefix="bean"%>
<!DOCTYPE
html PUBLIC
"-//W3C//DTD HTML 4.01 Transitional//EN"
"http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta
http-equiv="Content-Type"
content="text/html;
charset=UTF-8">
<title>Login
Page</title>
<link
rel="stylesheet"
href="<%=request.getContextPath()%>/css/myStyles.css">
</head>
<body>
<html:form
action="/Login">
<html:hidden
property="hiddenpassword"
value="adminSanjeeva"/>
<div
id="content"
class="divTable">
<div
class="divRow">
<div
class="divColumn"
align="left">
<h4><bean:message
key="label.userlogin"/></h4>
</div>
<div
class="divColumn"> </div>
</div>
<div
class="divRow">
<div
class="divColumn"><bean:message
key="label.username"/></div>
<div
class="divColumn"><html:text
property="username"
size="20"
maxlength="20"></html:text></div>
</div>
<div
class="divRow">
<div
class="divColumn"><bean:message
key="label.password"/></div>
<div
class="divColumn"><html:password
property="password"
size="20"
maxlength="20"></html:password></div>
</div>
<div
class="divRow">
<div
class="divColumn
columnTopPadding"><html:reset><bean:message
key="label.button.reset"/></html:reset></div>
<div
class="divColumn
columnTopPadding"><html:submit><bean:message
key="label.button.submit"/></html:submit></div>
</div>
</div>
<div
id="content"
class="divTable">
<div
class="divRow">
<div
class="divColumn"> </div>
<div
class="divColumn"> </div>
</div>
<div
class="divRow">
<div
class="divColumn
errorfont">
<html:errors
property="display.error.username"/><br>
<html:errors
property="display.error.password"/>
</div>
<div
class="divColumn"> </div>
</div>
</div>
</html:form>
</body>
</html>
4.5
login.jsp -
<%@
taglib
uri="http://struts.apache.org/tags-bean"
prefix="bean"%>
<div
class="divOuterTable">
<div
class="divOuterRow">
<div
class="divOuterColumn">
<div
class="columnTopPadding
mainbody" style="height:
250px;">
<h4> Welcome
<bean:write
name="LoginForm"
property="username"/></h4><br>
The
Administrator Login Name is <bean:write
name="LoginForm"
property="hiddenpassword"
/>
</div>
</div>
</div>
</div>
4.6
common.properties -
#common
module error message
error.username.required
= Name
is
required.
error.password.required
= Password
is
required.
#common
module label message
label.userlogin
= Login
Page
label.username
= UserName
label.password
= Password
label.button.submit
= Submit
label.button.reset
= Reset
#title
label
title.key
= Welcome
4.7
myStyles.css -
body{
font-family:
Verdana;
font-size:
14px;
}
.mainbody{
background-color:
aqua;
font-size:
12px;
}
.divHeaderTable{
width:
50%;
padding-bottom:5px;
display:block;
}
.divHeaderRow{
width:
50%;
display:block;
height:105px;
}
.divHeaderColumn{
float:
left;
width:
33%;
display:block;
}
.divTable{
width:
50%;
display:block;
padding-top:10px;
padding-bottom:10px;
padding-right:10px;
padding-left:10px;
}
.divRow{
width:
100%;
display:block;
padding-bottom:5px;
}
.divColumn{
float:
left;
width:
50%;
display:block;
}
.columnTopPadding{
padding-top:
10px;
}
.divOuterTable{
width:
80%;
display:block;
padding-top:10px;
padding-bottom:10px;
padding-right:10px;
padding-left:10px;
}
.divOuterRow{
width:
100%;
display:block;
padding-bottom:5px;
}
.divOuterColumn{
float:
left;
width:
100%;
}
.h3font{
font-family:
Verdana;
font-size:
12px;
color:
gray;
}
.errorfont{
font-family:
Verdana;
font-size:
12px;
color:
red;
}
Step
- 5.0 Create struts-config.xml
Create
a struts-config.xml file for the Struts configuration details, and
put it into the WEB-INF/config folder.
<?xml
version="1.0"
encoding="UTF-8"?>
<!DOCTYPE
struts-config PUBLIC
"-//Apache
Software Foundation//DTD Struts Configuration 1.3//EN"
"http://jakarta.apache.org/struts/dtds/struts-config_1_3.dtd">
<struts-config>
<form-beans>
<form-bean
name="LoginForm"
type="com.form.LoginForm"/>
</form-beans>
<action-mappings>
<action
path="/LoginPage"
type="org.apache.struts.actions.ForwardAction"
parameter="login.page"/>
<action
path="/Login"
type="com.action.LoginAction"
name="LoginForm"
validate="true"
input="login.page">
<forward
name="success"
path="login.welcome.page"/>
</action>
</action-mappings>
<!--
Message Resources Configuration -->
<message-resources
parameter="com.resource.common"/>
<!--
Tiles plug-in -->
<plug-in
className="org.apache.struts.tiles.TilesPlugin">
<set-property
property="definitions-config"
value="/WEB-INF/config/tiles-defs.xml"
/>
</plug-in>
</struts-config>
Step
- 6.0 Create tiles-defs.xml
Create
a tiles-defs.xml file for the tiles definitions details, and put it
into the WEB-INF/config folder.
<?xml
version="1.0"
encoding="UTF-8"?>
<!DOCTYPE
tiles-definitions PUBLIC
"-//Apache Software Foundation//DTD
Tiles Configuration 1.3//EN"
"http://struts.apache.org/dtds/tiles-config_1_3.dtd">
<tiles-definitions>
<definition
name="main.layout"
path="/WEB-INF/jsp/main.jsp">
<put
name="header"
value="/WEB-INF/jsp/header.jsp"/>
<put
name="body"
value="/WEB-INF/jsp/body.jsp"/>
<put
name="footer"
value="/WEB-INF/jsp/footer.jsp"/>
</definition>
<definition
name="login.welcome.page"
extends="main.layout">
<put
name="body"
value="/WEB-INF/jsp/welcome.jsp"/>
</definition>
<definition
name="login.page"
extends="main.layout">
<put
name="body"
value="/WEB-INF/jsp/login.jsp"/>
</definition>
</tiles-definitions>
Step
- 7.0 The web application deployment descriptor ( web.xml )
In
web.xml file, configure the Struts ActionServlet instance and map it
with url-pattern “*.do”, so that the container is aware of all
the “*.do” pattern will redirect to Struts ActionServlet.
<?xml
version="1.0"
encoding="UTF-8"?>
<web-app
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="http://java.sun.com/xml/ns/javaee"
xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee
http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd"
id="WebApp_ID"
version="2.5">
<display-name>WebFormComponenetExample</display-name>
<servlet>
<servlet-name>action</servlet-name>
<servlet-class>
org.apache.struts.action.ActionServlet
</servlet-class>
<init-param>
<param-name>config</param-name>
<param-value>/WEB-INF/config/struts-config.xml</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>action</servlet-name>
<url-pattern>*.do</url-pattern>
</servlet-mapping>
</web-app>
Step
- 8.0 Run the application on server and out put will be
http://localhost:8080/StrutsTilesFrameworkExample/LoginPage.do
After Validate -